Components
Textfield
Used to enter spesific text input form
Component Status Details
Status component contains a list of checks and completeness that has been tested and owned by each component
Textfields allow user input. The border should light up simply and clearly indicating which field the user is currently editing.
We have basic types of textfield:
- Basic Text Field
- Basic Text Field Outline
- Text Field With Label inline
- Text Field With Label Outline
and then states of textfield:
- Normal State Inline
- Normal State Outline
- Error State Inline
- Error State Outline
- Success State Inline
- Succes State Outline
- Disable State Inline
- Disable State Outline
You can also implement prefix and suffix for your project.
For textfield customization:
- Prefix Text Inline
- Prefix Text Outline
- Suffix Text Inline
- Suffix Text Outline
- Left Icon Inline Text Field
- Left Icon Outline Text Field
- Right Icon Inline Text Field
- Right Icon Outline Text Field
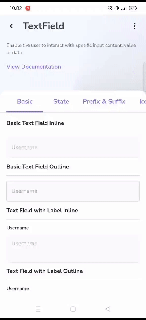
Usage
Basic
Textfield Inline
var value by remember { mutableStateOf("") }LgnInlineTextField(value = value,onValueChange = {value = it},placeholder = "Username")
Textfield Outline
var value by remember { mutableStateOf("") }LgnOutlineTextField(value = value,onValueChange = {value = it},placeholder = "Username")
With Label
Textfield with Inline Label
var value by remember { mutableStateOf("") }LgnFieldContainer(label = "Username") {LgnInlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",
Textfield with Outline Label
var value by remember { mutableStateOf("") }LgnFieldContainer(label = "Username") {LgnOutlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",
State
Normal State Inline Textfield
var value by remember { mutableStateOf("") }LgnFieldContainer(label = "Username") {LgnInlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",
Normal State Outline Textfield
var value by remember { mutableStateOf("") }LgnFieldContainer(label = "Username") {LgnOutlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",
Error State Inline Textfield
var value by remember { mutableStateOf("") }LgnFieldContainer(label = "Username", error = "Username Error") {LgnInlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",
Error State Outline Textfield
var value by remember { mutableStateOf("") }LgnFieldContainer(label = "Username", error = "Username Error") {LgnOutlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",
Success State Inline Textfield
var value by remember { mutableStateOf("") }LgnFieldContainer(label = "Username", success = "Username Correct") {LgnInlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",
Success State Outline Textfield
var value by remember { mutableStateOf("") }LgnFieldContainer(label = "Username", success = "Username Correct") {LgnOutlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",
Disabled State Inline Textfield
var value by remember { mutableStateOf("") }LgnInlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",singleLine = false,
Disabled State Outline Textfield
var value by remember { mutableStateOf("") }LgnOutlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",singleLine = false,
Prefix Text
Prefix Inline Textfield
var value by remember { mutableStateOf("") }LgnInlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",prefix = {
Prefix Outline Textfield
var value by remember { mutableStateOf("") }LgnOutlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",prefix = {
Suffix Text
Suffix Inline Textfield
var value by remember { mutableStateOf("") }LgnInlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",suffix = {
Suffix Outline Textfield
var value by remember { mutableStateOf("") }LgnOutlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",suffix = {
Left Icon
Left Icon Inline Textfield
var value by remember { mutableStateOf("") }LgnInlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",iconLeft = {
Left Icon Outline Textfield
var value by remember { mutableStateOf("") }LgnOutlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",iconLeft = {
Right Icon
Right Icon Inline Textfield
var value by remember { mutableStateOf("") }LgnInlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",iconRight = {
Right Icon Outline Textfield
var value by remember { mutableStateOf("") }LgnOutlineTextField(value = value,onValueChange = {value = it},placeholder = "Username",iconRight = {
Attributes
Parameters | Type | Description |
---|---|---|
value | String | Set Text in Field |
onValueChange | (String) -> Unit | Set Action When Text Change |
colors | LgnTextFieldColors | Set Color of Text |
textStyle | TextStyle | Set Text Style |
enabled | Boolean | Set Enable or Disable of Text Field |
error | Boolean | Set whether Text Field State Error or Not |
success | Boolean | Set whether Text Field State Success or Not |
iconLeft | @Composable (() -> Unit)? | Set Icon Left of Text Field |
iconRight | @Composable (() -> Unit)? | Set Icon Right of Text Field |
prefix | @Composable (() -> Unit)? | Set Prefix of Text Field |
suffix | @Composable (() -> Unit)? | Set Suffix of Text Field |
shape | Shape | Set Shape of Text Field |
placeHolder | String | Set Place Holder of Text Field |
keyboardOptions | KeyboardOptions | Set Options Available on the Keyboard when They Appear on the Screen |
keyboardActions | KeyboardOptions | What the Application Should Do after the User has Finished Entering Text |
singleLine | Boolean | Set The Text Field Whether Single Line or Not |
maxLines | Int | Set Maximum Line of Text Field |
minLines | Int | Set Minimum Line of Text Field |
visualTransformation | VisualTransformation | Changing Visual Output of the Text Field |