Components
Textfield
Used to enter spesific text input form
Status component contains a list of checks and completeness that has been tested and owned by each component
Usage
The Base Text Field component in the Legion iOS UIKit provides two types of fields: Outline and Inline. Each type of field has five states (default, focused, error, success, disabled) and supports additional features such as left icons, labels (title text field), captions, prefixes, and suffixes.
Importing The Theme
To use the Legion iOS UIKit theme, you need to import one of the available themes. Currently supported themes are as follows: ThemeAGR
, ThemeEazy
, ThemeIHS
, ThemeLGN
, and ThemeMyTEnS
.
import ThemeLGN
Style
Inline

let textField = LGNTextField()textField.style = .inline
Outline

let textField = LGNTextField()textField.style = .outline
Size
The LGNTextField offers three size variants: small (.sm
), medium (.md
), and large (.lg
).
Large
Inline

let textField = LGNTextField()textField.size = .lgtextField.style = .inline
Outline

let textField = LGNTextField()textField.size = .lgtextField.style = .outline
Medium
Inline

let textField = LGNTextField()textField.size = .mdtextField.style = .inline
Outline

let textField = LGNTextField()textField.size = .mdtextField.style = .outline
Small
Inline

let textField = LGNTextField()textField.size = .smtextField.style = .inline
Outline

let textField = LGNTextField()textField.size = .smtextField.style = .outline
State
The LGNTextField offer five state variants ranging from Default
, Focused
, Error
, Success
, and Disabled
.
Default
Inline

let textField = LGNTextField()textField.setState(.default)textField.style = .inline
Outline

let textField = LGNTextField()textField.setState(.default)textField.style = .outline
Focused
Inline

let textField = LGNTextField()textField.setState(.focused)textField.style = .inline
Outline

let textField = LGNTextField()textField.setState(.focused)textField.style = .outline
Error
Inline

let textField = LGNTextField()textField.setState(.error)textField.style = .inline
Outline

let textField = LGNTextField()textField.setState(.error)textField.style = .outline
Success
Inline

let textField = LGNTextField()textField.setState(.success)textField.style = .inline
Outline

let textField = LGNTextField()textField.setState(.success)textField.style = .outline
Disabled
Inline

let textField = LGNTextField()textField.setState(.disabled)textField.style = .inline
Outline

let textField = LGNTextField()textField.setState(.disabled)textField.style = .outline
Icon
Left Icon
Inline

let textField = LGNTextField()textField.leftIconSelect = UIImage(systemName: "envelope.fill")textField.style = .inline
Outline

let textField = LGNTextField()textField.leftIconSelect = UIImage(systemName: "envelope.fill")textField.style = .outline
Right Icon
Inline

let textField = LGNTextField()textField.rightIconSelect = UIImage(systemName: "xmark.circle.fill")textField.style = .inline
Outline

let textField = LGNTextField()textField.rightIconSelect = UIImage(systemName: "xmark.circle.fill")textField.style = .outline
Prefix
No Icon
Inline

let textField = LGNTextField()textField.textPrefix = "Prefix"textField.style = .inline
Outline

let textField = LGNTextField()textField.textPrefix = "Prefix"textField.style = .outline
With Left Icon
Inline

let textField = LGNTextField()textField.textPrefix = "Prefix"textField.leftIconSelect = UIImage(systemName: "envelope.fill")textField.style = .inline
Outline

let textField = LGNTextField()textField.textPrefix = "Prefix"textField.leftIconSelect = UIImage(systemName: "envelope.fill")textField.style = .outline
Suffix
No Icon
Inline

let textField = LGNTextField()textField.textSuffix = "Suffix"textField.style = .inline
Outline

let textField = LGNTextField()textField.textSuffix = "Suffix"textField.style = .outline
With Right Icon
Inline

let textField = LGNTextField()textField.textSuffix = "Suffix"textField.rightIconSelect = UIImage(systemName: "xmark.circle.fill")textField.style = .inline
Outline

let textField = LGNTextField()textField.textSuffix = "Suffix"textField.rightIconSelect = UIImage(systemName: "xmark.circle.fill")textField.style = .outline
Secure Text
Inline

let textField = LGNTextField()textField.isSecure = truetextField.style = .inline
Outline

let textField = LGNTextField()textField.isSecure = truetextField.style = .outline
Label
Label is an additional title for Textfield.
Inline
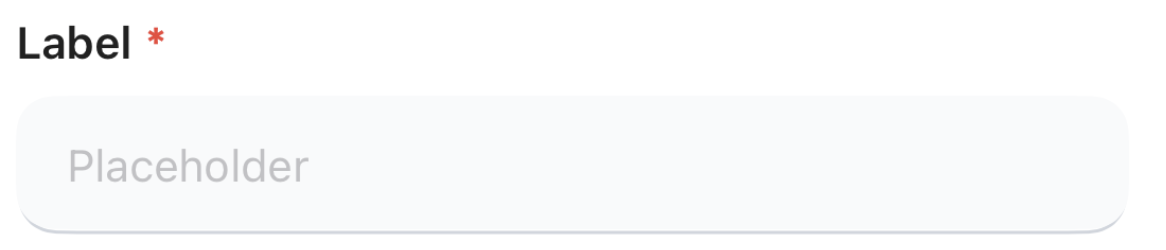
let textField = LGNTextField()textField.label(isHidden: false,textLabel: "Label",required: true)textField.style = .inline
Outline

let textField = LGNTextField()textField.label(isHidden: false,textLabel: "Label",required: true)textField.style = .outline
Caption
With Image
Inline
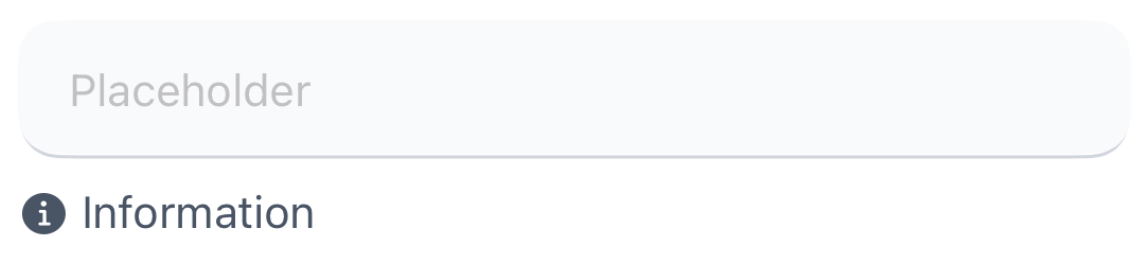
let textField = LGNTextField()textField.caption(isHidden: false,icon: UIImage(systemName: "info.circle.fill"),caption: "Information",iconColor: UIColor(hex: "475467"),textColor: UIColor(hex: "475467"))textField.style = .inline
Outline
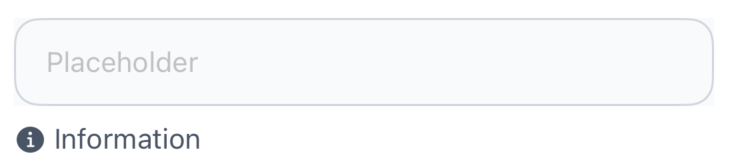
let textField = LGNTextField()textField.caption(isHidden: false,icon: UIImage(systemName: "info.circle.fill"),caption: "Information",iconColor: UIColor(hex: "475467"),textColor: UIColor(hex: "475467"))textField.style = .outline
Without Image
Inline

let textField = LGNTextField()textField.caption(isHidden: false,caption: "Information",textColor: UIColor(hex: "475467"))textField.style = .inline
Outline
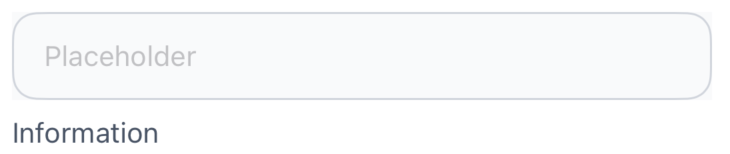
let textField = LGNTextField()textField.caption(isHidden: false,caption: "Information",textColor: UIColor(hex: "475467"))textField.style = .outline
Hint
Inline
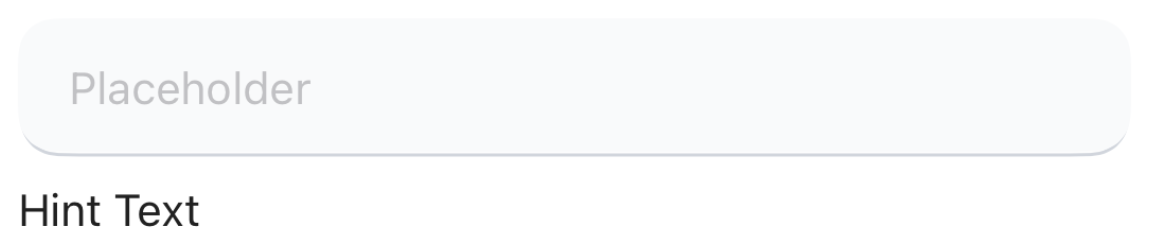
let textField = LGNTextField()textField.hintText(isHidden: false,textHint: "Hint Text")textField.style = .inline
Outline

let textField = LGNTextField()textField.hintText(isHidden: false,textHint: "Hint Text")textField.style = .outline
Full Configuration
Inline
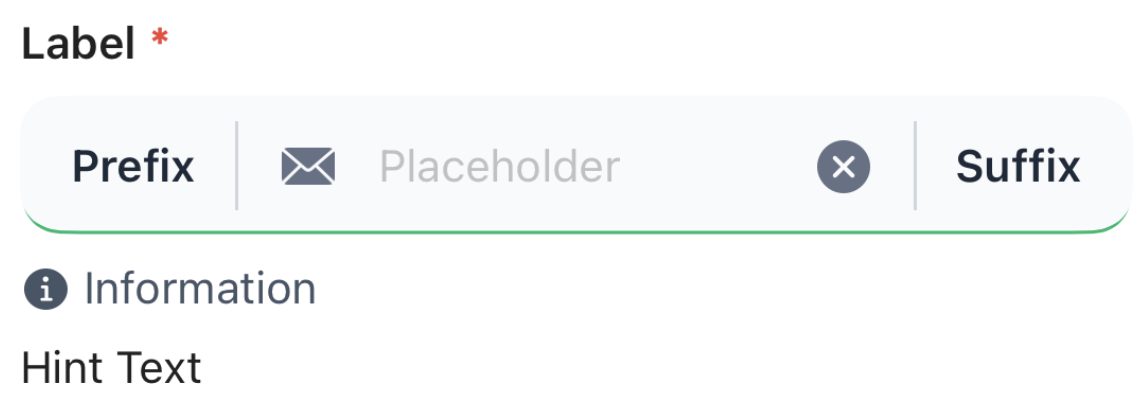
let textField = LGNTextField()textField.size = .mdtextField.label(isHidden: false,textLabel: "Label",required: true)textField.textPrefix = "Prefix"textField.leftIconSelect = UIImage(systemName: "envelope.fill")
Outline
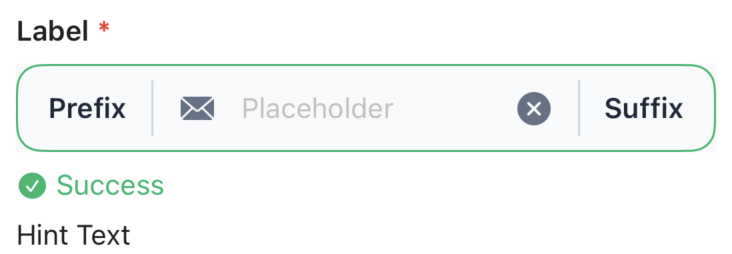
let textField = LGNTextField()textField.size = .mdtextField.label(isHidden: false,textLabel: "Label",required: true)textField.textPrefix = "Prefix"textField.leftIconSelect = UIImage(systemName: "envelope.fill")